Combining the elegance of Laravel’s framework with WordPress’s battle-tested content management is a fantastic method of getting a tech stack that delivers both developer efficiency and content creator freedom.
This guide shows you three powerful ways to integrate these platforms:
- A Laravel backend powering a WordPress frontend for robust application logic
- WordPress as a headless CMS for Laravel applications, giving you full frontend control
- A microservices architecture that lets both platforms shine in their specialized roles
Read ahead to find out how to architect solutions that leverage the best of both worlds without compromising on performance, security, or scalability.
Can you use WordPress and Laravel together?
Absolutely! The marriage of WordPress and Laravel is not only possible but can also result in a powerful and flexible online presence. This combination allows you to leverage the strengths of both platforms: the user-friendly content management of WordPress and the robust, developer-friendly features of Laravel.
Understanding WordPress and Laravel
WordPress
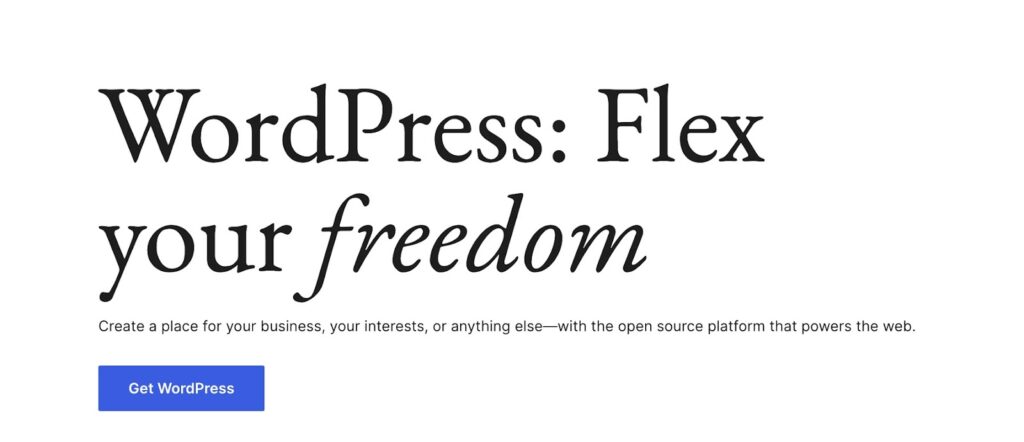
A leading CMS, WordPress powers 43.1% of the internet’s websites. Its strength lies in its:
- Ease of use and user-friendliness, even for non-technical individuals.
- Simplicity and flexibility in creating, managing, and publishing content.
- Ability to create a wide array of websites, including blogs, corporate websites, and eCommerce platforms.
- Open-source nature and vast ecosystem of themes and plugins, allowing developers to extend its functionality and customize websites to meet specific needs.
Laravel
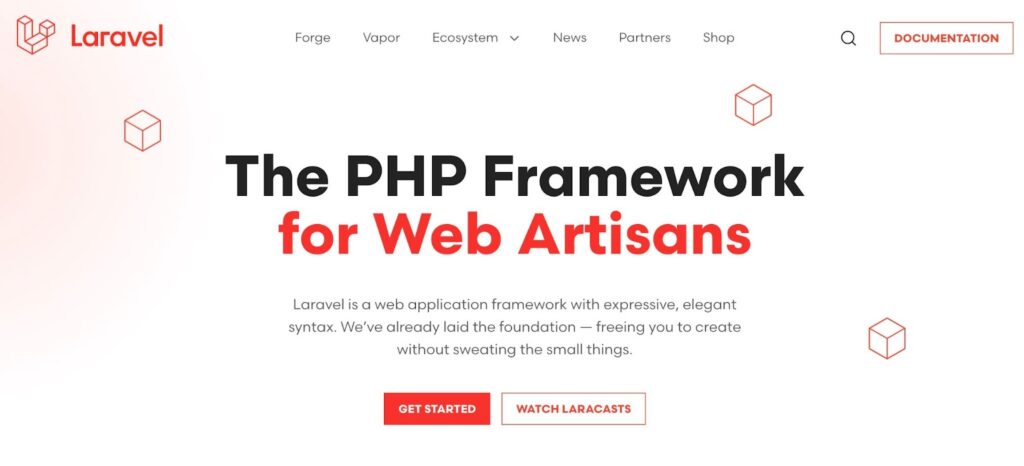
Laravel is an open-source PHP web application framework that provides an elegant and expressive syntax for web development. It follows the Model-View-Controller (MVC) architectural pattern, which separates the application logic into three main components: models (representing data and business logic), views (handling presentation and user interface), and controllers (managing the interaction between models and views).
The MVC architectural pattern promotes clean, organized code, making it a top choice for building complex web applications. This is accomplished by a host of features that streamline web application development, such as:
- A simple and flexible routing system to define how incoming requests are handled. This helps in creating clean and organized URLs for your application.
- Eloquent object-relational mapping (ORM), which is Laravel’s implementation of the active record pattern, allows programmers to work with databases using a fluent and intuitive syntax. It simplifies tasks like database querying, relationship management, and data manipulation.
- Blade Templating engine for easy-to-create dynamic and reusable views. Blade Templates are compiled into plain PHP code for efficient rendering.
- Artisan command-line tool that provides a set of pre-built commands to assist with various development tasks, such as creating controllers, models, migrations, and more.
- Built-in authentication and authorization mechanisms, making it straightforward to implement user registration, login, and access control for different parts of your application.
- Database seeding and migration systems that help in managing database schema changes over time. Additionally, database seeding enables you to populate your database with test data.
- Event-driven architecture, allowing you to define events and listeners to respond to those events.
- Broadcasting to facilitate real-time communication using technologies like WebSockets.
- API support that can be used to build both traditional web applications and RESTful APIs, making it suitable for building web services and mobile applications.
TL;DR? Here’s a quick comparison of both:
Aspect | WordPress | Laravel |
Primary use case | Content management system (CMS). | PHP web application framework. |
Ease of use | Extremely user-friendly, suited for non-developers. | Requires programming knowledge and experience. |
Flexibility | Highly customizable with themes and plugins. | Customizable architecture, following the MVC pattern. |
Content management | Specialized for content creation and management. | Focused on building robust web applications. |
Community support | Large and active community. | Active and growing community. |
Themes and plugins | Extensive library of themes and plugins available. | More focused on developer-oriented packages. |
SEO friendliness | Offers SEO-friendly features out of the box. | SEO optimization requires more manual configuration. |
Performance | May require optimization for heavy traffic. | Offers high performance with proper coding practices. |
Scalability | Suited for smaller to medium-sized websites. | Scales well for larger and more complex applications. |
Development speed | Quick setup and content creation. | Slightly longer development cycle due to complexity. |
Customization | Limited custom application development. | Allows for full-fledged custom application creation. |
Security | May require additional security plugins. | Strong security features and practices. |
Learning curve | Low barrier to entry, minimal coding knowledge. | Steeper learning curve and requires programming skills. |
Updates and maintenance | Simplified updates and maintenance. | May require more complex updates and maintenance. |
Benefits of using WordPress and Laravel together
This powerful duo can yield a myriad of benefits, as highlighted by Codeable expert Ismail Shehab, who has worked with world-class clients such as BMW Group, Rolls Royce, and The United Nations.
Speed and performance
Pairing WordPress with Laravel can result in a swift and high-performing website due to Laravel’s:
- Modular architecture, which allows for building web applications by creating components, which can significantly improve the speed of your site.
- Built-in caching mechanisms, such as file caching and support for popular caching services like Memcached and Redis, which can enhance your site’s performance.
- Use of Composer to optimize code execution that gives your website an additional performance boost.
Enhanced security
Laravel plays a major role in the terms of enhancing security because it offers:
- Robust security features that can fortify your website. For instance, Laravel provides cross-site request forgery (CSRF) protection through tokens, ensuring the authenticity of requests and preventing unwanted actions. It’s akin to having a secret handshake to verify the legitimacy of user requests.
- Eloquent ORM that uses prepared statements and parameter binding, making your database queries resistant to SQL injection attacks.
- Input validation and sanitization, which is key to preventing security issues like cross-site scripting (XSS) attacks or code injection.
- One-command system authentication, giving you extra security features such as secure password hashing (using algorithms like bcrypt or Argon2), encryption, and two-factor authentication.
Easier code maintenance
Laravel’s clean syntax makes coding much faster, and easier to read and maintain. For instance, you can type a singular command which will then create your entire authentication system for you, complete with login, registration, and dashboard UI.
This ease of use can significantly speed up your development process.
More resources to work with
Both Laravel and WordPress have large, active communities that offer countless resources, tutorials, and plugins to help you overcome challenges.
As they are both open-source, they are constantly evolving and improving. This means you’ll always have access to the latest features and best practices, making your development process smoother and more efficient.
How to combine WordPress and Laravel
By integrating WordPress into a Laravel application, you can create a website that boasts the best of both worlds: a dynamic web application with powerful functionalities, alongside a user-friendly content management system that simplifies content updates and modifications.
In essence, the fusion of WordPress and the Laravel framework represents a sophisticated interplay of technological prowess and creative vision. The choice of integration method depends on project goals, technical expertise, and the desired user experience. Here are three approaches that you can consider.
Approach 1: WordPress as the frontend and Laravel as the API backend
In this setup, WordPress serves as the frontend and CMS, while Laravel functions as the backend API. WordPress will manage and display your website’s content; meanwhile, Laravel can handle custom application logic, data processing, and database interactions.
WordPress offers a plethora of themes and plugins that simplify the process of creating responsive designs, optimizing performance, and enhancing user engagement. Laravel, in the backend, allows developers to build APIs that deliver data to the frontend seamlessly with its elegant syntax, modular structure, and comprehensive set of tools.
You can extend WordPress using the WordPress REST API (or the WooCommerce REST API) to expose the necessary endpoints for communication with the Laravel backend.
Approach 2: Laravel as the frontend and WordPress as the headless CMS
For this approach, Laravel will be the primary framework for building your website’s frontend. You can use Laravel’s Blade Templating engine and routing system to create the desired frontend views.
This method best suits those who want more flexibility and control over the frontend for specific design or functionality requirements.
Meanwhile, WordPress will act as a headless CMS (or headless WooCommerce ecosystem), used solely for content management and storage. You can retrieve content from WordPress using its REST API and consume it within your Laravel application. This can be achieved by leveraging packages like Corcel or WordPlate, which provide an easy way to use WordPress as a backend for Laravel.
Approach 3: Microservices architecture
For more complex requirements, you might consider adopting a microservices architecture. This approach treats WordPress and Laravel as separate applications, each serving specific functionalities.
For instance, you might use WordPress for content management and Laravel for custom application logic and data processing.
You can use inter-service communication mechanisms like REST APIs or message queues to allow the two systems to interact and share data. This architecture can provide a high degree of flexibility and scalability, as each microservice can be developed, deployed, and scaled independently.
Ultimately, the best approach depends on your specific needs and objectives. By carefully considering the strengths and weaknesses of WordPress and Laravel, you can create a web solution that delivers the best possible results for your business goals.
Manual integration vs. hiring a developer
Combining WordPress with Laravel is a complex task that requires a deep understanding of both platforms. Depending on your technical proficiency and the scope of your project, you may choose to undertake this task yourself or hire a professional developer based on the following considerations.
Manual integration
This approach requires a solid grasp of both platforms, as well as knowledge of PHP, databases, and web development best practices since you’ll be setting up WordPress and Laravel yourself.
While this might be a viable option for experienced developers, it can be a daunting task for those with limited technical skills or those who prefer to focus on other aspects of their project. It’s a complex process that can lead to detrimental hindrances if not done properly, such as:
- Increased development time.
- Debugging difficulties.
- Performance bottlenecks and potential conflicts between the two systems.
- Security vulnerabilities.
- Dealing with conflicting dependencies, libraries, or plugins that both platforms rely on.
Hiring a developer
Given the technical complexity of integrating WordPress with Laravel, hiring a developer can be a wise choice. A professional developer can provide the necessary expertise, ensure adherence to best practices, and deliver a robust and efficient solution.
This is where Codeable proves to be an excellent solution!
Codeable is a platform that connects you with top-tier WordPress developers for short-term and one-off projects. With a pool of over 700+ developers experienced in all areas of WordPress and WooCommerce, Codeable ensures you find the right expert for your project.
After submitting your project, you’ll be matched with 1-5 developers within a few hours and get a quote for your project which is an average of all the developers’ estimates. You’ll then be able to choose the right developer after chatting with them individually.
To get an idea of the level of expertise and professionalism a Codeable developer can deliver, consider the experience of Ismail Shehab. He recently built a custom loyalty program for a client’s WordPress eCommerce site using Laravel that included:
- Developing a Laravel API to handle points calculation and redemption logic, setting up models, migrations, and relationships for storing customer points and loyalty program rules.
- Connecting the client’s WordPress site to the Laravel API using a custom plugin and modifying their theme’s functions.php file.
- Using WooCommerce hooks to trigger API requests for points calculation and redemption whenever a customer made a purchase or redeemed points.
Consequently, Ismail successfully added unique functionality to the client’s site without modifying the WordPress core or the WooCommerce plugin. This approach not only maintained the site’s integrity but also offered the flexibility needed to create tailored features for the client’s customers.
Incorporating WordPress into your Laravel site manually
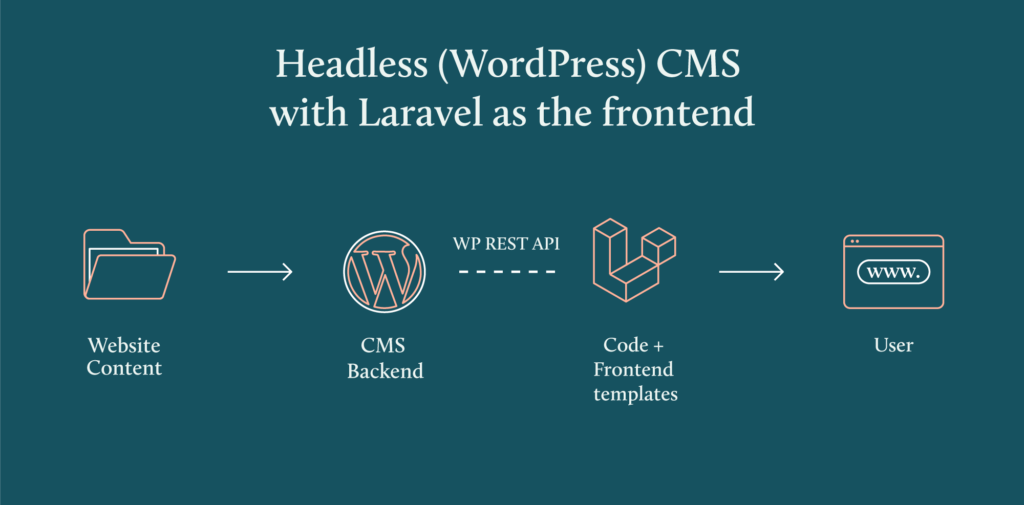
While the method you choose to fuse both platforms depends on your project’s specific requirements and preferences, here are detailed implementations for each approach. The actual implementation will need to be tweaked according to your exact requirements, but these guides will give you a solid foundation to build upon.
Laravel as the API backend approach
In this setup, WordPress handles the frontend while Laravel powers the backend API. Here’s how to implement it:
1. Set up WordPress frontend
- Install WordPress on your server
- Choose and customize your theme
- Install necessary plugins for frontend functionality
- Configure WordPress settings and permalinks
2. Create Laravel API backend
bash
composer create-project --prefer-dist laravel/laravel your-api-backend
cd your-api-backend
3. Set up API endpoints in Laravel
PHP
// routes/api.php
Route::prefix('api/v1')->group(function () {
Route::get('/data', 'ApiController@getData');
Route::post('/process', 'ApiController@processData');
});
4. Create API Controller
bash
php artisan make:controller ApiController
5. Implement API functionality
PHP
// app/Http/Controllers/ApiController.php
public function getData()
{
// Your data processing logic here
return response()->json([
'success' => true,
'data' => $processedData
]);
}
6. Connect WordPress to Laravel API
- Create a custom WordPress plugin to handle API communication
- Use WordPress HTTP API to make requests to Laravel endpoints
- Handle responses and update WordPress content accordingly
Headless CMS approach
This approach uses Laravel for the frontend while WordPress serves as a headless CMS. Here’s the implementation:
1. Install WordPress
- Set up WordPress installation
- Configure headless mode settings
- Define custom post types and fields
- Input your content
2. Install Laravel
bash
composer create-project --prefer-dist laravel/laravel your-project-name
cd your-project-name
3. Build Laravel frontend
- Create Blade templates for your views
- Set up routing structure
- Implement frontend controllers
4. Configure WordPress API access
PHP
// config/wordpress.php
return [
'base_url' => env('WP_API_URL'),
'username' => env('WP_API_USERNAME'),
'password' => env('WP_API_PASSWORD'),
];
5. Create content retrieval service
PHP
// app/Services/WordPressService.php
class WordPressService
{
public function getPosts()
{
$response = Http::get(config('wordpress.base_url') . '/wp-json/wp/v2/posts');
return $response->json();
}
}
6. Implement caching layer
PHP
// app/Http/Controllers/ContentController.php
public function index()
{
return Cache::remember('wp_posts', 3600, function () {
return $this->wordPressService->getPosts();
});
}
The microservices architecture approach
This approach treats WordPress and Laravel as separate services that communicate via APIs. Here’s how to implement it:
1. Set up service discovery
- Install and configure service registry (e.g., Consul)
- Register both WordPress and Laravel services
2. Configure message queue
bash
composer require php-amqplib/php-amqplib
3. Implement service communication
PHP
// app/Services/MessageQueue.php
class MessageQueue
{
public function publishMessage($exchange, $message)
{
$connection = new AMQPStreamConnection(
config('queue.host'),
config('queue.port'),
config('queue.user'),
config('queue.password')
);
$channel = $connection->channel();
$channel->exchange_declare($exchange, 'direct', false, true, false);
$channel->basic_publish($message, $exchange);
$channel->close();
$connection->close();
}
}
4. Create service interfaces
PHP
// app/Interfaces/ContentServiceInterface.php
interface ContentServiceInterface
{
public function fetchContent($type);
public function updateContent($type, $data);
}
5. Implement health checks
PHP
// routes/api.php
Route::get('/health', function () {
return response()->json(['status' => 'healthy']);
});
6. Set up monitoring
- Configure logging aggregation
- Implement distributed tracing
- Set up alerting system
Remember to implement proper error handling, logging, and monitoring for each approach. Also, ensure you’re following security best practices such as:
- Implementing proper authentication between services
- Using HTTPS for all API communications
- Sanitizing data at both ends
- Implementing rate limiting
- Setting up proper CORS policies
- Using secure environment variables for sensitive data
The choice between these approaches depends on factors such as:
- Scale requirements
- Performance needs
- Development team expertise
- Maintenance capabilities
- Budget constraints
- Time to market requirements
Best practices for working with WordPress and Laravel
It’s imperative to follow best practices to ensure the smooth operation of your website. The following tips are meticulously curated by Codeable expert Ismail Shehab to help you maintain a secure, efficient, and high-performing site:
- Separate files and directories: Separate your Laravel and WordPress files and directories so you can update each platform independently without affecting the other. For instance, you can place your Laravel files in a subdirectory of your WordPress installation.
- Keep both platforms up-to-date: Both WordPress and Laravel frequently release updates that address vulnerabilities, introduce new features, and improve stability. Therefore, it’s important to keep your WordPress versions, Laravel packages, WP plugins, themes, and PHP up-to-date.
- Regular backups: Creating regular backups of your site ensures that you have a safety net in case something goes wrong, saving you from potential data loss or site downtime. This can be done automatically with plugins like UpdraftPlus or manually from cPanel or FTP. Laravel also offers backup packages for added convenience.
- Test updates on a staging environment: Before applying updates to your live site, it’s highly recommended to test them on a staging environment first. A staging environment is a replica of your live website where you can test updates, plugins, themes, or any changes without impacting your live site.
- Use version control systems: Version control systems like Git are invaluable tools for managing your codebase. They allow you to track changes effectively and efficiently, and roll back to previous versions if needed. They’re also useful for collaborating with other developers on your team.
Consider hiring a professional for maintenance tasks
Maintaining a WordPress and Laravel site requires technical expertise, especially when it comes to security, performance optimization, and troubleshooting. Consider hiring a professional developer who specializes in WordPress and Laravel. Codebale’s experts can:
- Ensure the proper functioning of your websites.
- Identify and fix any bugs.
- Handle updates.
- Implement security measures.
- Optimize performance.
- Save you time and effort.
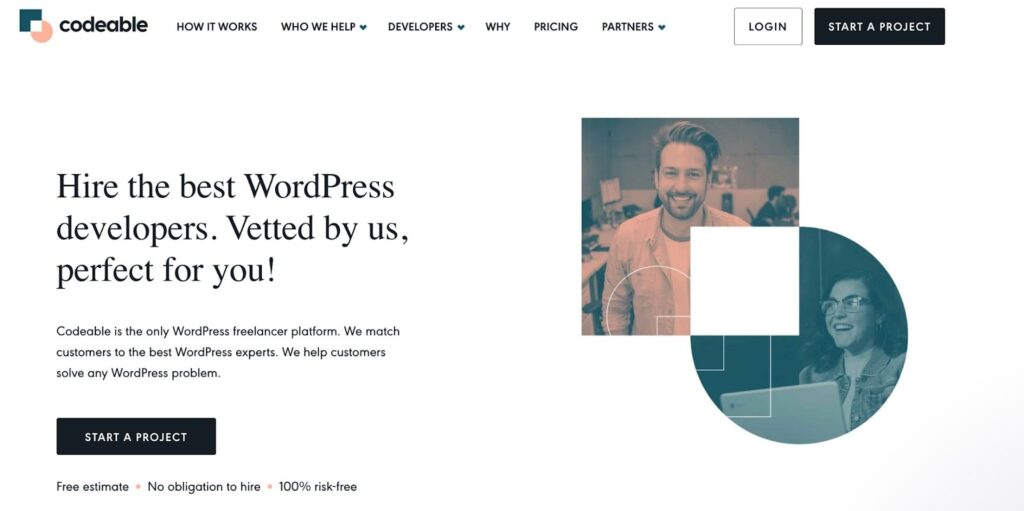
Remember that each project might have unique conditions, so adapt these best practices to suit your specific demands. Regularly review your approach to ensure that it aligns with the evolving best practices of both the WordPress and Laravel communities.
Incorporate WordPress into your Laravel website with Codeable
WordPress and Laravel integration isn’t just a technical possibility – it’s a game-changing strategy for building modern, scalable websites. By combining WordPress’s content management prowess with Laravel’s development flexibility, you’re not just building a website; you’re creating a foundation for digital excellence.
Whether you choose to implement the backend API approach, go headless with WordPress, or embrace a microservices architecture, the possibilities are endless. And while the technical complexity might seem daunting, platforms like Codeable connect you with experts who can bring your vision to life.
Ready to revolutionize your web presence? Submit your project to Codeable today with an expert who can guide you through the journey. The future of web development is here – and it speaks both WordPress and Laravel fluently.
The post How to Incorporate WordPress Into Your Laravel Site appeared first on Codeable.